Python time.sleep() – How to Make a Time Delay in Python
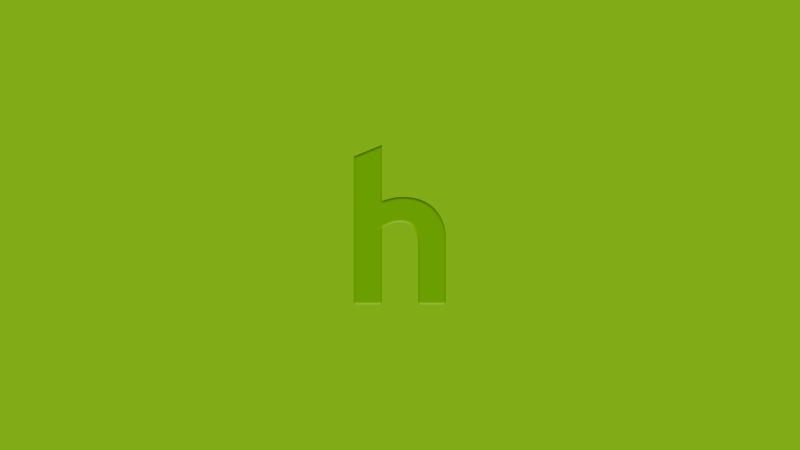
Share
What you'll need
Interests
Learn how to use Python’s sleep function to make a timed delay.
tl;dr
import time
time.sleep(seconds)
1 – Import the time module
We will first want to import Python’s time module:
import time
This module ships with Python so it should be available on any system. Although it is not necessary for this guide, I highly recommend getting familiar with the time module. Read more about the time module here.
2 – Use time.sleep to set the delay
You can set a delay in your Python script by passing the number of seconds you want to delay to the sleep function:
time.sleep(5)
This means you want the script to delay 5 seconds before continuing. The sleep function also accepts floats if you want to give a more precise number:
time.sleep(1.75)
This will sleep for 1 second and 750 milliseconds. You can also use a float to delay for less than a second like this:
time.sleep(0.5)
This will sleep for half of a second. You can and should read more about Python’s sleep function here.