How to Turn an Object into Query String Parameters in JavaScript
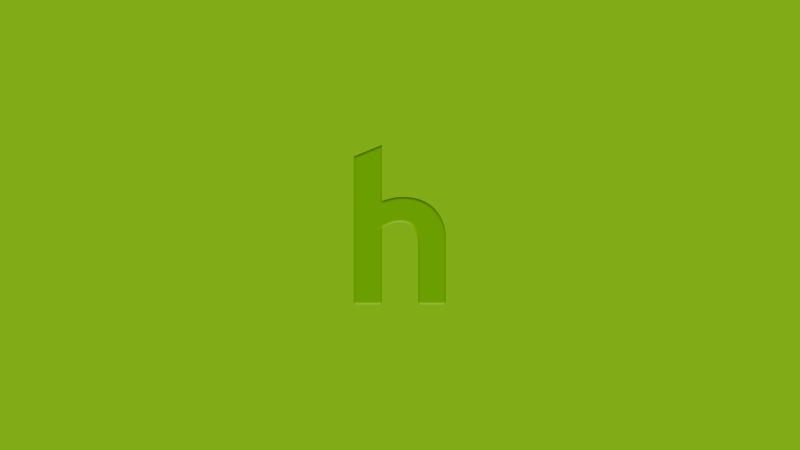
Share
Interests
As a JavaScript developer, you’ll often need to construct URLs and query string parameters. One sensible way to construct query string parameters is to use a one layer object with key value pairs.
In this guide we’ll cover various ways to turn an object like this:
var params = {
a: 1,
b: 2,
c: 3
};
into a query string like this:
"a=1&b=2&c=3"
1 – Using map and join
If you’re using a modern browser (or node) you can use map
to create an array of strings like a=1
, then use join
to join them together with &
.
ES6
var queryString = Object.keys(params).map(key => key + '=' + params[key]).join('&');
ES5
var queryString = Object.keys(params).map(function(key) {
return key + '=' + params[key]
}).join('&');
2 – Using jQuery
If you’re the sort of person who uses jQuery, you’ve got a solution readily available:
var queryString = $.param(params);
3 – Using the querystring module in node
If you’re using node, you can use the querystring module:
const querystring = require('querystring');
let queryString = querystring.stringify(params);
4 – Parameter encoding
If you know you’re keys and values need to be encoded, you should use encodeURIComponent
like this:
var queryString = Object.keys(params).map((key) => {
return encodeURIComponent(key) + '=' + encodeURIComponent(params[key])
}).join('&');
It’s also possible to query by timestamp in MySQL.