How to Pretty Print JSON in Chrome Developer Console
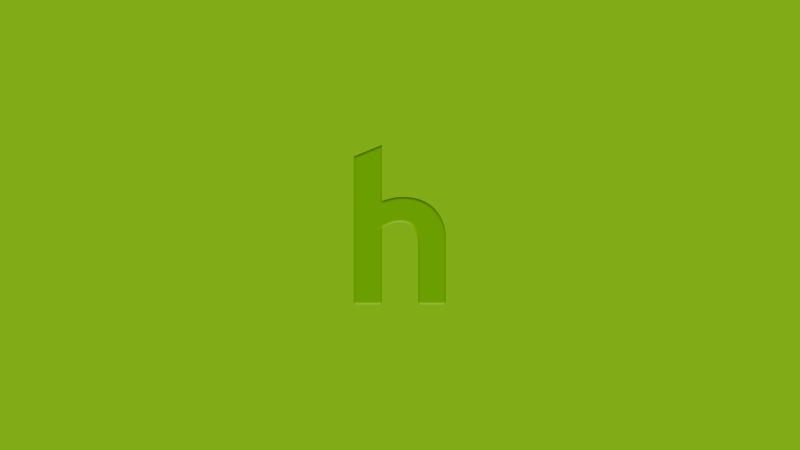
Share
Interests
Posted in these interests:
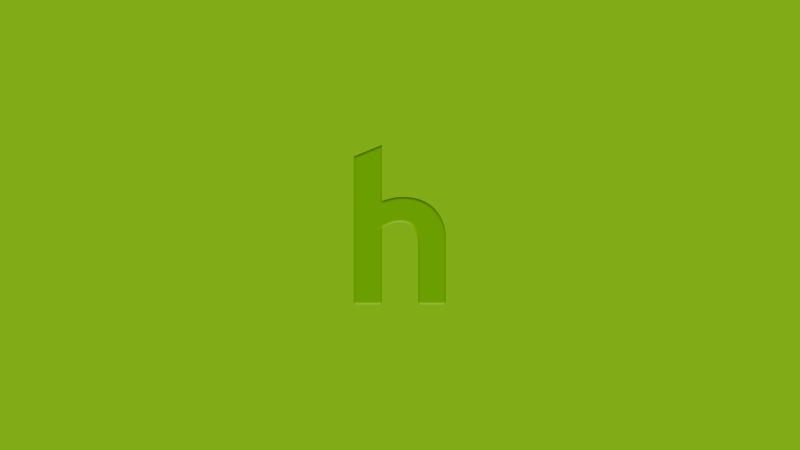
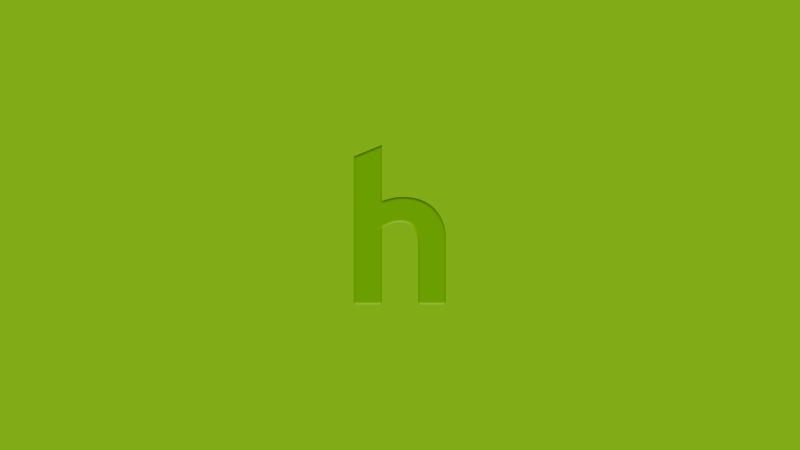
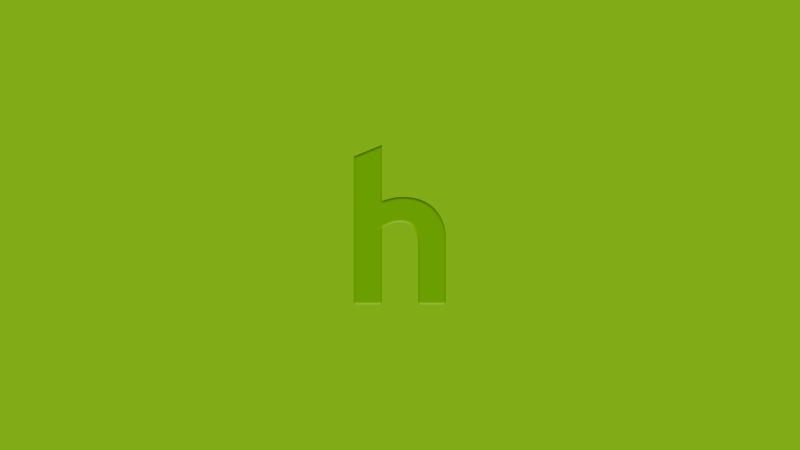
This short guide will show you how to pretty print a JSON object in the Chrome Developer Tools console.
1 – Output your object as a string
Wrap your object in JSON.stringify
and log it to the console:
const currentlyDrinking = {
beer: 'Yeungling',
container: 'bottle',
empty: true
};
console.log(JSON.stringify(currentlyDrinking));
This will result in the following minified object:
{"beer":"Yeungling","container":"bottle","empty":true}
Not exactly pretty yet, so we’ll need to format it. This is especially important for larger objects.
2 – Format the pretty-printed output
To format our object, we’ll need to specify the number of spaces we’d like:
const currentlyDrinking = {
beer: 'Yeungling',
container: 'bottle',
empty: true
};
console.log(JSON.stringify(currentlyDrinking, undefined, 4)); // use 4-space tabs to format and indent the code
This results in:
{
"beer": "Yeungling",
"container": "bottle",
"empty": true
}
Much better!
3 – More formatting options
For more Chrome Developer console object pretty-printing options, check out the JSON.stringify() MDN docs.