Secure Your Sensitive Data with Kubernetes Secrets
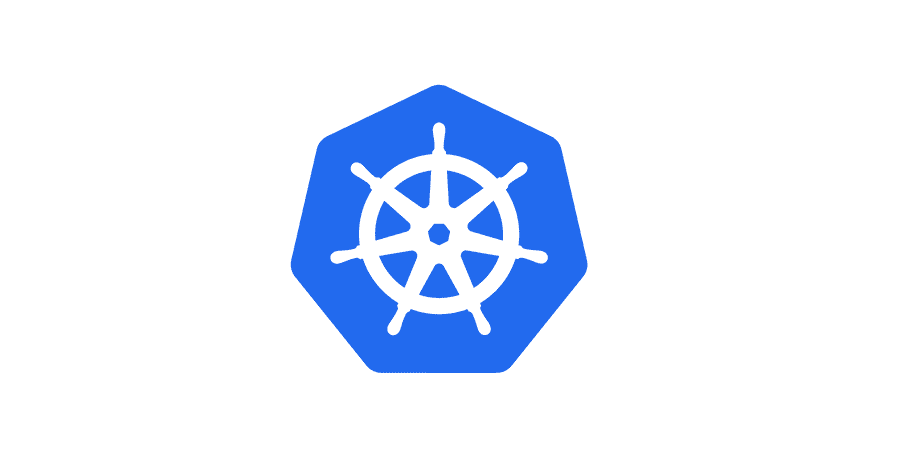
Share
Interests
Posted in these interests:
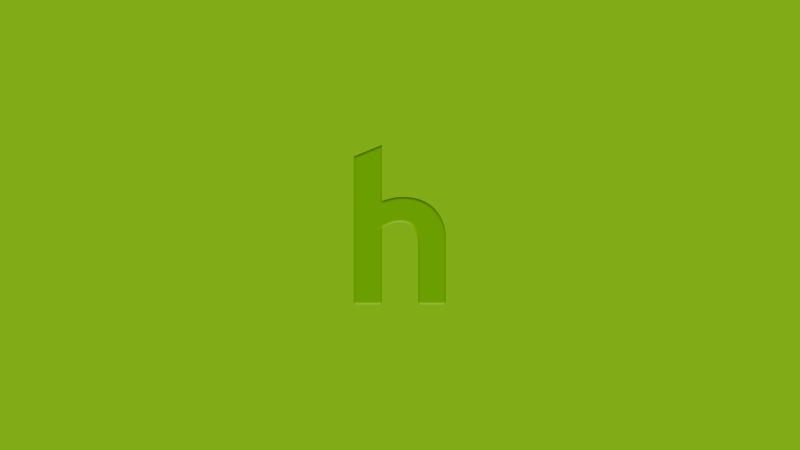
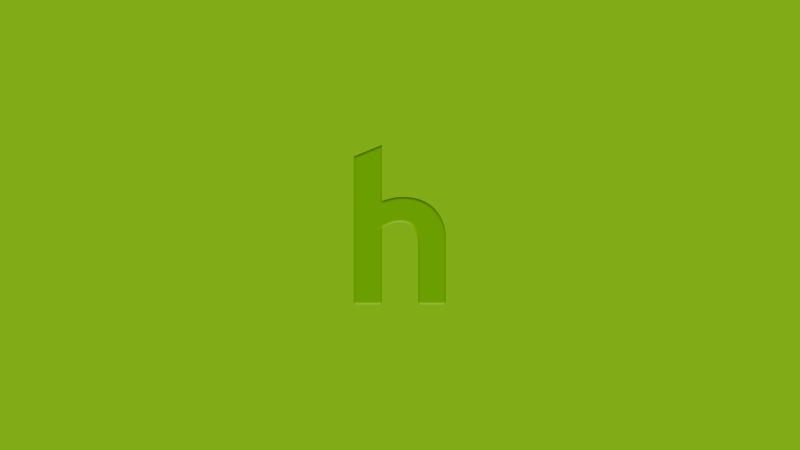
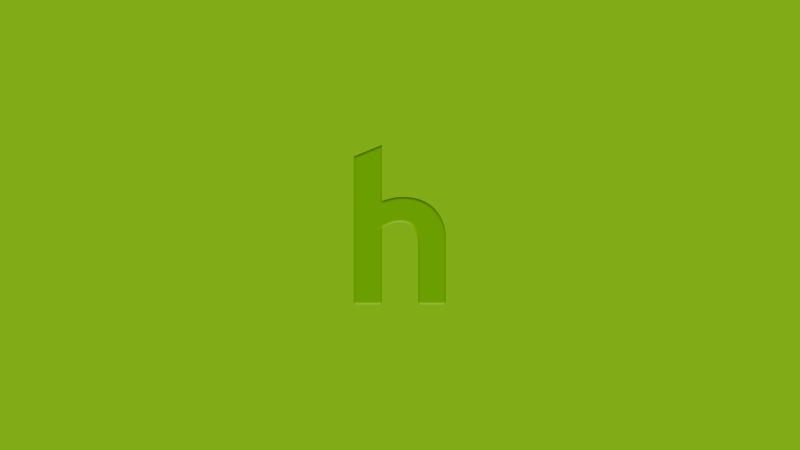
Kubernetes secrets
are objects that store and manage sensitive data inside your Kubernetes cluster. One mistake developers often make is storing sensitive information like database passwords, API credentials, etc in a settings file in their codebase. This is very bad practice (hopefully for obvious reasons). Most developers know this, but still choose the option because it’s easy.
Fortunately, if you’re running your application in a Kubernetes cluster, managing that sensitive data the right way is easy. This guide will provide an overview to Kubernetes Secrets, including how to create, store, and use secrets in your applications.
1 – Create a Kubernetes secret
Creating secrets, like most Kubernetes operations, is accomplished using the kubectl
command. Fortunately, there are a few ways to create secrets, and each are useful in different circumstances.
Let’s first look at the secret we want to create. Remember that the secret is an object that contains one or more pieces of sensitive data. For our example, let’s imagine we want to create a secret, called database
, that contains our database credentials. It will be constructed like this:
database
- username
- password
Create a secret from files
Suppose you have the following files: username
and password
. They might have been created like this:
echo -n 'databaseuser' > ./username
echo -n '1234567890' > ./password
We can use these files to construct our secret:
kubectl create secret generic database --from-file=./username --from-file=./password
Create a secret from string literals
If you’d prefer, you can skip the files altogether and create the secret from string literals:
kubectl create secret generic database --from-literal=username=databaseuser --from-literal=password=databaseuser
Examine the new secret
Both of the above examples will create identical secrets that look like this:
$ kubectl get secret database
NAME TYPE DATA AGE
database Opaque 2 1h
And let’s example the secret:
$ kubectl describe secret database
Name: database
Namespace: default
Labels: <none>
Annotations:
Type: Opaque
Data
====
username: 12 bytes
password: 10 bytes
Copy secrets from another cluster or namespace
While this is directly applicable, I’ll add this as a note because it could be useful. Sometimes we’ll need to copy secrets from one cluster or namespace to another. Here’s a quick example:
kubectl get secret database --context source_context --export -o yaml | kubectl apply --context destination_context -f -
For an explanation and more details, see our guide on copying Kubernetes secrets from one cluster to another.
2 – Attach secrets to your pod
Secrets aren’t all that helpful until they’re attached to a pod. In order to actually use the secrets they must be configured in the pod definition.
There are two primary ways two use secrets: as files and as environment variables.
Attaching secrets as files
See the following pod config:
apiVersion: v1
kind: Pod
metadata:
name: web
spec:
containers:
- name: web
image: web:1.0.0
volumeMounts:
- name: database-volume
mountPath: "/etc/secrets/database"
readOnly: true
volumes:
- name: database-volume
secret:
secretName: database
There are two important blocks to take note of. First, let’s look at the volumes
block. We set the name of the volume and specify which secret we want to use. Note that this is set at the pod
level, so it could be used in multiple containers if the pod were to define them.
volumes:
- name: database-volume
secret:
secretName: database
Next we’ll look at how the volume is mounted onto the container using volumeMounts
. We’ll specify which volume we want to use, and set the mount path to /etc/secrets/database
.
volumeMounts:
- name: database-volume
mountPath: "/etc/secrets/database"
readOnly: true
Inside of the container, we can run an ls
on /etc/secrets/database
and find that both the username
and password
files exist.
Attaching secrets as environment variables
Secrets can also be used inside of containers as environment variables. Check out the same config but with secrets attached as environment variables instead of volumes:
apiVersion: v1
kind: Pod
metadata:
name: web
spec:
containers:
- name: web
image: web:1.0.0
env:
- name: DATABASE_USERNAME
valueFrom:
secretKeyRef:
name: database
key: username
- name: DATABASE_PASSWORD
valueFrom:
secretKeyRef:
name: database
key: password
Summary
Both volumes and environment variables are perfectly acceptable ways to access secrets from inside your containers. The major difference is that environment variables can only hold a single value, while volumes can hold any number of files—even nested directories. So if your application requires access to many secrets, a volume is a better choice for organization and to keep the configs manageable.
3 – Accessing secrets from within the container (using Python)
I know some readers will not be using Python containers, but the purpose of this step is to provide a conceptual understanding of how secrets can be used from within the container.
Assuming you’ve followed the first two steps, you should now have a database
secret that contains a username
and password
.
Reading secrets from a volume
If we’ve mounted the secret as a volume, we can read the secret like this:
with open('/etc/secrets/database/password, 'r') as secret_file:
database_password = secret_file.read()
Grabbing the secret file is as easy reading from a file. Of course, you’d probably abstract this code and add error handling and defaults. After all, this is much more pleasant: get_secret('database/password')
.
Reading secrets from environment variables
This is even more straight forward, at least in Python. You can read the secret just as you would any other environment variable:
import os
database_password = os.environ.get('DATABASE_PASSWORD')
4 – Conclusion
I hope this overview of Kubernetes secrets was helpful. By now, you should have a good understand of what Kubernetes secrets are and how to use them. If you have questions, please ask in the comments below or head over to the Kubernetes secrets documentation.